Forms II - they're back, and they're not happy...
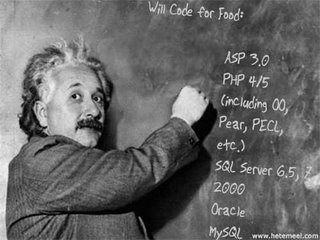
When we created our first form we used a php script to list all of the global variables - useful for testing the form, but not much good if we want to actually use the information for something. This week we're going to look at how to get individual form elements into variables so we can bend them to our will.
Setting up
To start off make sure you've given the text area, checkbox and menu form elements meaningful name attributes:
- name = "shipname" for the first text box
- name = "tripdate" for the second
- name = "exploration" for the first checkbox
- name = "contact" for the second
- name = "species" for the multiselect dropdown menu
To process the form we'll need to create a new php page to process our form: testform.php
This will be a common, or garden variety html page, but buried in the <body> section we need to add a block of php code:
<?php
// set a variable from the shipname field on the form...
$shipname = $_POST["shipname"];
print("Thank you $shipname.");
?>
This code looks for an input control on the form called: shipname and assigns it to a php variable: $shipname, it then writes this variable and a quick thank you on the screen.
Update the form so that it's action="testform.php", and upload both files. Don't forget to set the testform.php file permissions to 'execute' so that the web server can run it.
Debug if necessary and once you have it working, try adding code to display the tripdate and exploration elements.
Being Selective
Did you notice how the exploration control returned a value (perhaps something like 'yes')? What we want to do is try some selection on this value before we write the expedition objectives on the screen. Something like:
print("<strong>Mission Goals:</strong> <br />");
$exploration = $_POST["exploration"];
if ($exploration == "yes") {
print("Exploration<br />");
};
Try adding this code to your own page. You might need to change the comparison string to match the value attribute of your form's checkbox. Once you get this working, add code for showing 'Contact' if the contact check box was selected.
An optional extra:
The final part of the form is a bit trickier. The crew species list can have more than one value selected. This will be returned as an array of values to the php form checking page, and so we need to do a bit of fancy php footwork to access it.
Firstly, we need to give the option control a php array-friendly name. If its currently called: name="species" you will need to add the array delimiters to its name, making it: name="species[]" - you should now have html on your form page which looks something like:
<select name="species[]" size="4" multiple>
In your php file you need to run a for loop through this array, printing each value on the screen:
print("<p><strong>Mission member's species:</strong><br />");
foreach($_POST['species'] as $species) {
print("$species <br/>");
}
print("</p>");
This takes the species array from the post array and itereates through it, assigning each selected species value to a $species variable, which it prints on the screen. Simple really (actually, a bit more advanced that we're expecting from anyone just yet, but I put it in for the terminally curious).
Where to next? I think we'll be using a form for gathering feedback on a website sometime soon, perhaps even for the greening australia assignment, so it would be useful to think about what fields you'd put on this sort of page, and how you might retrieve that information once it was submitted to the server.
Bonus Section
Try adding some radio buttons for the ultimate mission goal:
- inter-galactic domination or
- creation of a trade hedgonomy
Thanks to pvera for the picture: "Will code for food"
No comments:
Post a Comment